This simple script will use the LED's of the 3d-xmas-tree-for-raspberry-pi to indicate WiFi activity on the Raspberry Pi.
I have installed a default Raspbian with python.
Create the following script
from gpiozero import LEDBoard
from gpiozero.tools import random_values
from signal import pause
import time
import psutil
from decimal import *
tree = LEDBoard(*range(4,28),pwm=True)
star = LEDBoard(2,pwm=True)
netstats=psutil.net_io_counters(pernic=True)
totb = netstats['wlan0'].bytes_sent+netstats['wlan0'].bytes_recv
maxb = 0
def treelight(power):
for led in tree:
led.value=power
time.sleep(0.02)
for led in tree:
led.off()
def calc_power(delb):
getcontext().rounding = ROUND_CEILING
global maxb
p=float()
if delb > maxb:
maxb = delb
p = float(delb) / float(maxb)
if p > 0 and p < 0.1:
p = 0.09
return round(Decimal(p),1)
while True:
netstats=psutil.net_io_counters(pernic=True)
newb=netstats['wlan0'].bytes_sent+netstats['wlan0'].bytes_recv
delb=newb-totb
#print newb,totb,delb
if newb > totb:
treelight(calc_power(delb))
totb=newb
and save it as a Python script (/home/pi/wlan_monitor.py)
run it by executing:
python /home/pi/wlan_monitor.py
You will not see any output but the lights of the tree will blink when there is WiFi activity.
The intensity of the lights will depend on the amount of bytes transferred.
MySuSEproject
Just my 0.02€ on the OpenSuSE Distro
Wednesday, December 26, 2018
Sunday, December 31, 2017
Wordpress on OpenSuSE 42.3
First install the lamp pattern.
According to the Wordpress documentation you do not need additional php packages. However, after problems with the dashboard (internal server errorr 500) I followed the advice in this post and installed...
Now you can follow the Wordpress installation documentation.
sudo zypper in -t pattern lamp_server
According to the Wordpress documentation you do not need additional php packages. However, after problems with the dashboard (internal server errorr 500) I followed the advice in this post and installed...
sudo zypper in php5-zlib php5-xmlrpc php5-gd php5-ftp php5-curl
Now you can follow the Wordpress installation documentation.
Friday, May 5, 2017
dvdauthor does not accept hauppauge streams
Hauppauge streams can be used directly when you want to convert to other formats.
However, if you want to create a dvd with dvdauthor you might get the following errors:
To fix this you need to demux and mux (remultiplex) the file. This will add the needed NAV packets in the file
This will create a fixed.mpg file that you now can use with all tools
However, if you want to create a dvd with dvdauthor you might get the following errors:
DVDAuthor::dvdauthor, version 0.7.2. Build options: gnugetopt graphicsmagick iconv freetype fribidi Send bug reports to <dvdauthor-users@lists.sourceforge.net> INFO: default video format is PAL INFO: dvdauthor creating VTS STAT: Picking VTS 01 STAT: Processing 2017-04-02_10:43:05.mpg... WARN: Skipping sector at inoffset 0, waiting for first VOBU... WARN: Skipping sector at inoffset 0, waiting for first VOBU... WARN: Skipping sector at inoffset 0, waiting for first VOBU... WARN: Skipping sector at inoffset 0, waiting for first VOBU... WARN: Skipping sector at inoffset 0, waiting for first VOBU... WARN: Skipping sector at inoffset 0, waiting for first VOBU... WARN: Skipping sector at inoffset 0, waiting for first VOBU... WARN: Skipping sector at inoffset 0, waiting for first VOBU... WARN: Skipping sector at inoffset 0, waiting for first VOBU... WARN: Skipping sector at inoffset 0, waiting for first VOBU... WARN: Skipping sector at inoffset 0, waiting for first VOBU... WARN: Skipping sector at inoffset 0, waiting for first VOBU...
To fix this you need to demux and mux (remultiplex) the file. This will add the needed NAV packets in the file
sudo zypper in mjpegtools ProjectX projectx.sh -demux original.mpg -name fixed mplex -f 8 -o fixed.mpg fixed.m2v fixed.mp2
This will create a fixed.mpg file that you now can use with all tools
Wednesday, August 31, 2016
Use Raspberry Pi + HVR1900 to record set-top box output (Telenet digicorder) and stream to DLNA devices
Technically not an OpenSuSE post, I know!
date: Sept. 2016
What you need:
- Raspberry Pi (I'm using a first generation model B rev 2 with 512MB RAM) and power supply
- SD card
- Hauppauge HVR-1900 connected to set-top box (Composite cables to SCART conversion plug)
- External USB drive
1. Install Raspbian on SD card
Go to https://www.raspberrypi.org/downloads/raspbian/ and download the Raspbian Jessie Lite (almost everything you need is already included in this image)Follow the already well documented instructions to write this image to an SD card (and make sure you use a working card)
Make sure the external devices (HDD and HVR-1900) are already connected BEFORE powering on your Pi!
I'm working headless so all I need is the IP address of the Pi after it boots. This page can help you.
Use an SSH-client to connect to the ip address with username "pi" and default password "raspberry".
Now start the built-in configuration assistant to configure basic settings (more info here):
pi@raspberrypi:~ $ sudo raspi-config
┌─────────┤ Raspberry Pi Software Configuration Tool (raspi-config) ├──────────┐ │ │ │ 1 Expand Filesystem Ensures that all of the SD card s │ │ 2 Change User Password Change password for the default u │ │ 3 Boot Options Choose whether to boot into a des │ │ 4 Wait for Network at Boot Choose whether to wait for networ │ │ 5 Internationalisation Options Set up language and regional sett │ │ 6 Enable Camera Enable this Pi to work with the R │ │ 7 Add to Rastrack Add this Pi to the online Raspber │ │ 8 Overclock Configure overclocking for your P │ │ 9 Advanced Options Configure advanced settings │ │ 0 About raspi-config Information about this configurat │ │ │ │ │ │ <Select> <Finish> │ │ │ └──────────────────────────────────────────────────────────────────────────────┘
- change the user PASSWORD (option 2)
- set the correct LOCALE (option 5, sub option 1, nl_BE.UTF-8 UTF-8 in my case)
- set the correct TIMEZONE (option 3, sub option 2, Europe/Brussels in my case)
pi@raspberrypi:~ $ sudo apt-get update pi@raspberrypi:~ $ sudo apt-get upgrade pi@raspberrypi:~ $ sudo reboot
2. Configure HVR-1900
The HVR-1900 is not working out of the box. The drivers and the utilities are already loaded (pvrusb2 module and v4l2-utils are included in the raspbian distribution) but we are still missing the firmware.Let's check the kernel ring buffer messages for relevant output:
pi@raspberrypi:~ $ dmesg | grep -i pvrusb2 [ 11.104384] pvrusb2: Hardware description: WinTV HVR-1900 Model 73xxx [ 11.118840] usbcore: registered new interface driver pvrusb2 [ 11.118874] pvrusb2: V4L in-tree version:Hauppauge WinTV-PVR-USB2 MPEG2 Encoder/Tuner [ 11.118890] pvrusb2: Debug mask is 31 (0x1f) [ 12.119585] usb 1-1.3: Direct firmware load for v4l-pvrusb2-73xxx-01.fw failed with error -2 [ 12.119629] pvrusb2: ***WARNING*** Device fx2 controller firmware seems to be missing. [ 12.119645] pvrusb2: Did you install the pvrusb2 firmware files in their proper location? [ 12.119660] pvrusb2: request_firmware unable to locate fx2 controller file v4l-pvrusb2-73xxx-01.fw [ 12.119674] pvrusb2: Failure uploading firmware1 [ 12.119685] pvrusb2: Device initialization was not successful. [ 12.119696] pvrusb2: Giving up since device microcontroller firmware appears to be missing. pi@raspberrypi:~ $
The HVR-1900 is a device that will load all necessary firmware when it is connected. To get it working you need the correct firmware files in the correct folder and retry the connection.
On some other linux distributions you have to download the appropriate files for your device (or extract them from the official windows drivers) and put them in the correct path (e.g. /lib/firmware)
So download this file and copy it to /lib/firmware
also install some other firmwares that are supplied in packages
pi@raspberrypi:~ $ sudo apt-get install firmware-ivtv pi@raspberrypi:~ $ sudo apt-get install firmware-linux-nonfree
Now that you have all the necessary files you can reload the driver by typing:
pi@raspberrypi:/lib/firmware $ sudo rmmod pvrusb2 pi@raspberrypi:/lib/firmware $ sudo modprobe pvrusb2
The dmesg command should now show something like this
pi@raspberrypi:/lib/firmware $ dmesg | tail -n 50 [ 2738.078101] usbcore: deregistering interface driver pvrusb2 [ 2738.078224] pvrusb2: Device being rendered inoperable [ 2744.193891] pvrusb2: Hardware description: WinTV HVR-1900 Model 73xxx [ 2744.196793] usbcore: registered new interface driver pvrusb2 [ 2744.196825] pvrusb2: V4L in-tree version:Hauppauge WinTV-PVR-USB2 MPEG2 Encoder/Tuner [ 2744.196841] pvrusb2: Debug mask is 31 (0x1f) [ 2745.205158] pvrusb2: Device microcontroller firmware (re)loaded; it should now reset and reconnect. [ 2745.400786] usb 1-1.3: USB disconnect, device number 5 [ 2745.401383] pvrusb2: Device being rendered inoperable [ 2747.172979] usb 1-1.3: new high-speed USB device number 6 using dwc_otg [ 2747.283021] usb 1-1.3: New USB device found, idVendor=2040, idProduct=7300 [ 2747.283059] usb 1-1.3: New USB device strings: Mfr=1, Product=2, SerialNumber=3 [ 2747.283079] usb 1-1.3: Product: WinTV [ 2747.283096] usb 1-1.3: Manufacturer: Hauppauge [ 2747.283115] usb 1-1.3: SerialNumber: 7300-00-F077FF16 [ 2747.288188] pvrusb2: Hardware description: WinTV HVR-1900 Model 73xxx [ 2747.321714] pvrusb2: Binding ir_rx_z8f0811_haup to i2c address 0x71. [ 2747.321941] pvrusb2: Binding ir_tx_z8f0811_haup to i2c address 0x70. [ 2747.360449] cx25840 3-0044: cx25843-24 found @ 0x88 (pvrusb2_a) [ 2747.373770] pvrusb2: Attached sub-driver cx25840 [ 2747.439531] tuner 3-0042: Tuner -1 found with type(s) Radio TV. [ 2747.439610] pvrusb2: Attached sub-driver tuner [ 2749.608253] cx25840 3-0044: loaded v4l-cx25840.fw firmware (16382 bytes) [ 2749.708553] tveeprom 3-00a2: Hauppauge model 73219, rev D2F5, serial# 4034395926 [ 2749.708589] tveeprom 3-00a2: MAC address is 00:0d:fe:77:ff:16 [ 2749.708608] tveeprom 3-00a2: tuner model is NXP 18271C2 (idx 155, type 54) [ 2749.708627] tveeprom 3-00a2: TV standards PAL(B/G) PAL(I) SECAM(L/L') PAL(D/D1/K) ATSC/DVB Digital (eeprom 0xf4) [ 2749.708644] tveeprom 3-00a2: audio processor is CX25843 (idx 37) [ 2749.708659] tveeprom 3-00a2: decoder processor is CX25843 (idx 30) [ 2749.708675] tveeprom 3-00a2: has radio, has IR receiver, has IR transmitter [ 2749.708720] pvrusb2: Supported video standard(s) reported available in hardware: PAL-B/B1/D/D1/G/H/I/K;SECAM-B/D/G/H/K/K [ 2749.708817] pvrusb2: Device initialization completed successfully. [ 2751.919944] cx25840 3-0044: loaded v4l-cx25840.fw firmware (16382 bytes) [ 2752.053610] tda829x 3-0042: setting tuner address to 60 [ 2752.105965] tda18271 3-0060: creating new instance [ 2752.153623] TDA18271HD/C2 detected @ 3-0060 [ 2752.673620] tda18271: performing RF tracking filter calibration [ 2771.814088] tda18271: RF tracking filter calibration complete [ 2771.884116] tda829x 3-0042: type set to tda8295+18271 [ 2775.682667] usb 1-1.3: Direct firmware load for v4l-cx2341x-enc.fw failed with error -2 [ 2775.682710] pvrusb2: ***WARNING*** Device encoder firmware seems to be missing. [ 2775.682725] pvrusb2: Did you install the pvrusb2 firmware files in their proper location? [ 2775.682739] pvrusb2: request_firmware unable to locate encoder file v4l-cx2341x-enc.fw [ 2775.683323] pvrusb2: registered device video0 [mpeg] [ 2775.683361] DVB: registering new adapter (pvrusb2-dvb) [ 2775.700154] pvrusb2: Clearing driver error statuss [ 2778.494356] cx25840 3-0044: 0x0000 is not a valid video input! [ 2778.552382] usb 1-1.3: DVB: registering adapter 0 frontend 0 (NXP TDA10048HN DVB-T)... [ 2778.563342] tda829x 3-0042: type set to tda8295 [ 2778.602153] tda18271 3-0060: attaching existing instance
Now do a quick test recording
Telenet digicorder specific (and maybe other set-top boxes): The scart output of the digicorder (DC-AD2100) has some quirks. In my case, the digicorder is connected to the TV using HDMI and to the HVR-1900 using scart-to-composite connectors. I found that you need to turn on the TV and watch the content you wish to record otherwise you only get sound. Also, make sure the audio volume of the digicorder is not too high because otherwise you get bad quality.
pi@raspberrypi:~ $ v4l2-ctl --set-input=1 # to activate the composite input Video input set to 1 (composite: ok) pi@raspberrypi:~ $ v4l2-ctl -s pal-B # because I live in a PAL-B standard country Standard set to 00000007 pi@raspberrypi:~ $ cat /dev/video0 > /home/pi/test.mpg
Press CTRL+C after a minute to stop recording...
Now get the file from your Pi (using an sftp client like filezilla) and check it with an MPEG capable player (like VLC). Since the scart output is interlaced you will need to activate the deinterlace function of you player to get rid of the horizontal lines during quick movement.
3. Automount the external drive
First, get the details of the connected devicepi@raspberrypi:~ $ sudo blkid /dev/mmcblk0p1: SEC_TYPE="msdos" LABEL="boot" UUID="22E0-C711" TYPE="vfat" PARTUUID="dad99d56-01" /dev/mmcblk0p2: UUID="202638e1-4ce4-45df-9a00-ad725c2537bb" TYPE="ext4" PARTUUID="dad99d56-02" /dev/sda1: LABEL="tosh1TB" UUID="ce555d59-e22f-4f0b-b8c2-9a77bc2a5374" TYPE="ext4" PARTUUID="148cf869-01"
I'm looking for a Toshiba drive so it is the last line. The UUID is the unique number of this drive that well remain the same across reboot or even on other machines.
Now we need to mount the device to a local folder for easy access. To do this you need to create a subfolder at /mnt.
pi@raspberrypi:~ $ sudo mkdir /mnt/usb pi@raspberrypi:~ $ sudo chown -R pi:pi /mnt/usb #to get correct permissions on that folder
Now edit the /etc/fstab file and add one line to the end. Press CTRL+X to exit and save the file:
pi@raspberrypi:~ $ sudo nano /etc/fstab
GNU nano 2.2.6 File: /etc/fstab proc /proc proc defaults 0 0 /dev/mmcblk0p1 /boot vfat defaults 0 2 /dev/mmcblk0p2 / ext4 defaults,noatime 0 1 # a swapfile is not a swap partition, no line here # use dphys-swapfile swap[on|off] for that UUID="ce555d59-e22f-4f0b-b8c2-9a77bc2a5374" /mnt/usb ext4 auto,users 0 0 ^G Get Help ^O WriteOut ^R Read File ^Y Prev Page ^K Cut Text ^C Cur Pos ^X Exit ^J Justify ^W Where Is ^V Next Page ^U UnCut Text^T To Spell
UUID is the unique ID for that disk
/mnt/usb is the mountpoint you want to use
ext4 is the filesystem type for this disk
auto,users are settings related for automount and security
0 0 is for dump and checkdisk settings
if you now reboot or issue this command...
pi@raspberrypi:~ $ sudo mount -a pi@raspberrypi:~ $ ls -ltr /mnt/usb total 28 drwx------ 2 pi users 16384 Jul 24 2013 lost+found drwxr-xr-x 2 pi users 4096 Oct 20 2013 tmp drwxr-xr-x 28 pi users 4096 Dec 30 2015 Maxtor drwxr-xr-x 2 pi users 4096 Aug 31 19:23 capture pi@raspberrypi:~ $
you can see that the contents of the disk are available at /mnt/usb.
I created a capture folder to keep all captures.
4. Remote control your setup (start/stop)
Our goal is to use a simple web interface to start and stop the recording so you do not need to use a SSH for this.To generate the web interface we will use the Flask framework because it's simple and also has a web server built-in.
Install it (and all dependencies) with:
pi@raspberrypi:~ $ sudo apt-get install python3-flask
It can take a while, afterward we need to create 2 files: the main python program and the html template.
NOTE: You will have to adapt this program because every HVR-1900 device has its own serial number. Only one line to adjust:
/sys/class/pvrusb2/sn-4034395926 <== change this number
pi@raspberrypi:~ $ nano /home/pi/pvr2web.py
now paste this text
#!/usr/bin/python3 import subprocess import datetime import time from flask import Flask from flask import render_template app = Flask(__name__)
@app.route('/pvr2web/', defaults={'action': None}, methods=['POST','GET']) @app.route('/pvr2web/<action>', methods=['POST','GET']) def process_action(action): if action == 'start': start='{:%Y-%m-%d_%H:%M:%S}'.format(datetime.datetime.now()) subprocess.call(["v4l2-ctl","--set-input=1"]) subprocess.call(["v4l2-ctl","-s","pal-B"]) subprocess.call("cat /dev/video0 > /mnt/usb/capture/" + start +".mpg &",shell=True) elif action =='stop': subprocess.call("killall cat",shell=True) time.sleep(1) stdoutdata = subprocess.getoutput("cat /sys/class/pvrusb2/sn-4034395926/ctl_streaming_enabled/cur_val") if stdoutdata == 'true': action = 'Recording' else: action = None return render_template('index2.html',action=action) if __name__ == '__main__': app.run(host='0.0.0.0',debug=True)
Press CTRL+X to exit and save, then run
pi@raspberrypi:~ $ chmod +x pvr2web.py #to make it executable
For the html template we need to create a subfolder
pi@raspberrypi:~ $ mkdir /home/pi/templates pi@raspberrypi:~ $ nano /home/pi/templates/index2.html
paste the following text
<!DOCTYPE html> <html> <head> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> h1 { text-align: center; background-color: lightgray; padding: 25px 25px; } h2 { color: lightgray; padding: 25px 25px; } .button { background-color: #4CAF50; border: none; color: white; padding: 15px 32px; text-align: center; text-decoration: none; display: inline-block; font-size: 24px; margin: 4px 4px; cursor: pointer; } .button2 {background-color: #f44336;} /* Red */ </style> </head> <body> <h1>Raspberry PI PVR</h1> <div style="text-align:center;"> {% if action %} <a href="/pvr2web/stop" class="button button2">Stop Recording</a> {% else %} <a href="/pvr2web/start" class="button">Start Recording</a> {% endif %} </div> </body> </html>
Press CTRL+X and save
Start the program to give it a try:
pi@raspberrypi:~ $ /home/pi/pvr2web.py * Running on http://0.0.0.0:5000/ * Restarting with reloader
Now go to a web browser and try it out: http://ip-address-of-pi:5000/pvr2web
You should see the following:
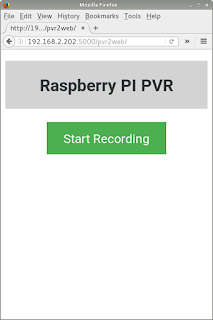
Now press the green button...
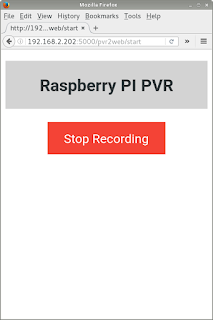
Press stop... This is what you should see in the console output
pi@raspberrypi:~ $ /home/pi/pvr2web.py * Running on http://0.0.0.0:5000/ * Restarting with reloader Video input set to 1 (composite: ok) Standard set to 00000007 192.168.2.116 - - [31/Aug/2016 19:53:30] "GET /pvr2web/start HTTP/1.1" 200 - 192.168.2.116 - - [31/Aug/2016 19:54:10] "GET /pvr2web/stop HTTP/1.1" 200 -
A file with a timestamp should now exist in the target path
pi@raspberrypi:~ $ ls -ltr /mnt/usb/capture/ total 30146560 -rw-r--r-- 1 pi pi 30146560 Aug 31 19:54 2016-08-31_19:53:29.mpg pi@raspberrypi:~ $
now we only have to autostart this program at boot time. We are going to use CRON for this because it is already installed. It just requires adding one line to a file.
pi@raspberrypi:~ $ crontab -e no crontab for pi - using an empty one /usr/bin/select-editor: 1: /usr/bin/select-editor: gettext: not found 'select-editor'. 1. /bin/ed /usr/bin/select-editor: 1: /usr/bin/select-editor: gettext: not found 2. /bin/nano <---- 3. /usr/bin/vim.tiny /usr/bin/select-editor: 32: /usr/bin/select-editor: gettext: not found 1-3 [2]: 2
and add this line at the bottom: @reboot /home/pi/pvr2web.py &
exit and save using CTRL+X and reboot...
5. Install minidlna (ReadyMedia) to stream to DLNA clients
install minidlna and change the library pathpi@raspberrypi:~ $ sudo apt-get install minidlna pi@raspberrypi:~ $ sudo nano /etc/minidlna.conf change "media_dir=/var/lib/minidlna" to "media_dir=/mnt/usb/capture"
Press CTRL + X to exit and save
Now restart the service
pi@raspberrypi:~ $ sudo systemctl restart minidlna
And try to connect using VLC
DONE!
Optional: use NFS to connect from *NIX clients.
NFS gives you the highest transfer between the pi and others.Installation is easy:
pi@raspberrypi:~ $ sudo apt-get install nfs-common nfs-kernel-server
configuration is done by adding 2 lines to a config file:
pi@raspberrypi:~ $ sudo nano /etc/exports
just add the 2 last lines and press CTRL+X to exit and save
GNU nano 2.2.6 File: /etc/exports Modified # /etc/exports: the access control list for filesystems which may be exported # to NFS clients. See exports(5). # # Example for NFSv2 and NFSv3: # /srv/homes hostname1(rw,sync,no_subtree_check) hostname2(ro,sync,no_sub$ # # Example for NFSv4: # /srv/nfs4 gss/krb5i(rw,sync,fsid=0,crossmnt,no_subtree_check) # /srv/nfs4/homes gss/krb5i(rw,sync,no_subtree_check) # /mnt/usb/ 192.168.2.0/24(rw,fsid=root,no_subtree_check) /mnt/usb/capture 192.168.2.0/24(rw,no_subtree_check,nohide) ^G Get Help ^O WriteOut ^R Read File ^Y Prev Page ^K Cut Text ^C Cur Pos ^X Exit ^J Justify ^W Where Is ^V Next Page ^U UnCut Text^T To Spell
Now reload NFS by issuing:
pi@raspberrypi:~ $ sudo exportfs -rav exporting 192.168.2.0/24:/mnt/usb/capture exporting 192.168.2.0/24:/mnt/usb
pi@raspberrypi:/mnt/usb/capture $ sudo systemctl enable rpcbind pi@raspberrypi:/mnt/usb/capture $ sudo systemctl start rpcbind pi@raspberrypi:/mnt/usb/capture $ sudo systemctl restart nfs-kernel-server
From another machine you can now connect using...
sudo mkdir /mnt/nfs sudo mount 192.168.2.202:/ /mnt/nfs
Sunday, March 24, 2013
No sound when recording with HVR-1900 after upgrading to openSuSE 12.3
I'm using a WinTV-HVR-1900 - Hauppauge Computer Works for analogue TV recording.
Using the same script for many years I was surprised to notice that all the new recordings did not contain any sound.
For a moment I thought the device was broken but after re-trying with openSuSE 12.2 I concluded that it had to be a software error or setting.
Using the command
This is the output for openSuSE 12.2 where everything works as expected:
[ 1438.650407] pvrusb2: ================= START STATUS CARD #0 =================
[ 1438.652688] cx25840 9-0044: Video signal: present
[ 1438.652693] cx25840 9-0044: Detected format: PAL-BDGHI
[ 1438.652697] cx25840 9-0044: Specified standard: automatic detection
[ 1438.652701] cx25840 9-0044: Specified video input: Composite 7
[ 1438.652704] cx25840 9-0044: Specified audioclock freq: 48000 Hz
[ 1438.658092] cx25840 9-0044: Detected audio mode: mono
[ 1438.658101] cx25840 9-0044: Detected audio standard: A2-BG
[ 1438.658108] cx25840 9-0044: Audio microcontroller: running
[ 1438.658115] cx25840 9-0044: Configured audio standard: automatic detection
[ 1438.658122] cx25840 9-0044: Configured audio system: automatic standard and mode detection
[ 1438.658132] cx25840 9-0044: Specified audio input: Tuner (In8)
[ 1438.658140] cx25840 9-0044: Preferred audio mode: stereo
[ 1438.658149] cx25840 9-0044: Selected 65 MHz format: system DK
[ 1438.658155] cx25840 9-0044: Selected 45 MHz format: chroma
[ 1438.667850] cx25840 9-0044: IR Receiver:
[ 1438.667869] cx25840 9-0044: Enabled: no
[ 1438.667881] cx25840 9-0044: Demodulation from a carrier: disabled
[ 1438.667894] cx25840 9-0044: FIFO: disabled
[ 1438.667905] cx25840 9-0044: Pulse timers' start/stop trigger: disabled
[ 1438.667917] cx25840 9-0044: FIFO data on pulse timer overflow: overflow marker
[ 1438.667929] cx25840 9-0044: FIFO interrupt watermark: half full or greater
[ 1438.667941] cx25840 9-0044: Loopback mode: normal receive
[ 1438.667958] cx25840 9-0044: Max measurable pulse width: 318144512 us, 318144512000 ns
[ 1438.667974] cx25840 9-0044: Low pass filter: disabled
[ 1438.667990] cx25840 9-0044: Pulse width timer timed-out: no
[ 1438.668026] cx25840 9-0044: Pulse width timer time-out intr: enabled
[ 1438.668038] cx25840 9-0044: FIFO overrun: no
[ 1438.668048] cx25840 9-0044: FIFO overrun interrupt: enabled
[ 1438.668064] cx25840 9-0044: Busy: no
[ 1438.668076] cx25840 9-0044: FIFO service requested: no
[ 1438.668089] cx25840 9-0044: FIFO service request interrupt: enabled
[ 1438.668101] cx25840 9-0044: IR Transmitter:
[ 1438.668111] cx25840 9-0044: Enabled: no
[ 1438.668123] cx25840 9-0044: Modulation onto a carrier: disabled
[ 1438.668135] cx25840 9-0044: FIFO: disabled
[ 1438.668147] cx25840 9-0044: FIFO interrupt watermark: half full or less
[ 1438.668159] cx25840 9-0044: Carrier polarity: space:noburst mark:burst
[ 1438.668174] cx25840 9-0044: Max pulse width: 318144512 us, 318144512000 ns
[ 1438.668187] cx25840 9-0044: Busy: no
[ 1438.668200] cx25840 9-0044: FIFO service requested: yes
[ 1438.668211] cx25840 9-0044: FIFO service request interrupt: enabled
[ 1438.668227] cx25840 9-0044: Brightness: 128
[ 1438.668244] cx25840 9-0044: Contrast: 68
[ 1438.668262] cx25840 9-0044: Saturation: 64
[ 1438.668279] cx25840 9-0044: Hue: 0
[ 1438.668296] cx25840 9-0044: Volume: 62225
[ 1438.668313] cx25840 9-0044: Mute: false
[ 1438.668331] cx25840 9-0044: Balance: 0
[ 1438.668350] cx25840 9-0044: Bass: 0
[ 1438.668365] cx25840 9-0044: Treble: 0
[ 1438.668384] pvrusb2: cx2341x config:
[ 1438.668396] pvrusb2: Stream: MPEG-2 Program Stream
[ 1438.668412] pvrusb2: VBI Format: No VBI
[ 1438.668424] pvrusb2: Video: 720x480, 30 fps
[ 1438.668438] pvrusb2: Video: MPEG-2, 4x3, Variable Bitrate, 6000000, Peak 8000000
[ 1438.668460] pvrusb2: Video: GOP Size 12, 2 B-Frames, GOP Closure
[ 1438.668475] pvrusb2: Audio: 48 kHz, MPEG-1/2 Layer II, 224 kbps, Stereo, No Emphasis, No CRC
[ 1438.668494] pvrusb2: Spatial Filter: Manual, Luma 1D Horizontal, Chroma 1D Horizontal, 0
[ 1438.668509] pvrusb2: Temporal Filter: Manual, 8
[ 1438.668522] pvrusb2: Median Filter: Off, Luma [0, 255], Chroma [0, 255]
[ 1438.668541] pvrusb2_a driver: <ok> <init> <connected> <mode=analog>
[ 1438.668555] pvrusb2_a pipeline: <idle> <configok>
[ 1438.668567] pvrusb2_a worker: <decode:quiescent> <encode:virgin> <encode:waitok> <usb:stop> <pathway:ok>
[ 1438.668581] pvrusb2_a state: ready
[ 1438.668597] pvrusb2_a Hardware supported inputs: television, dtv, composite, s-video; allowed inputs: television, composite, s-video
[ 1438.668622] pvrusb2_a Bytes streamed=0 URBs: queued=0 idle=0 ready=0 processed=0 failed=0
[ 1438.668636] pvrusb2_a ir scheme: id=2 Zilog
[ 1438.668650] pvrusb2_a Associated v4l2-subdev drivers and I2C clients:
[ 1438.668664] pvrusb2_a cx25840: cx25840 @ 44
[ 1438.668676] pvrusb2_a tuner: tuner @ 42
[ 1438.668686] pvrusb2: ================== END STATUS CARD #0 ==================
And this is the output for openSuSE 12.3... let's look for the difference!
[31341.497675] pvrusb2: ================= START STATUS CARD #0 =================
[31341.500021] cx25840 0-0044: Video signal: not present
[31341.500041] cx25840 0-0044: Detected format: NTSC-M
[31341.500053] cx25840 0-0044: Specified standard: automatic detection
[31341.500065] cx25840 0-0044: Specified video input: Composite 7
[31341.500077] cx25840 0-0044: Specified audioclock freq: 48000 Hz
[31341.515412] cx25840 0-0044: Detected audio mode: forced mode
[31341.515423] cx25840 0-0044: Detected audio standard: forced audio standard
[31341.515429] cx25840 0-0044: Audio microcontroller: detecting
[31341.515436] cx25840 0-0044: Configured audio standard: undefined
[31341.515443] cx25840 0-0044: Configured audio mode: MONO1 (LANGUAGE A/Mono L+R channel for BTSC, EIAJ, A2)
[31341.515449] cx25840 0-0044: Specified audio input: Tuner (In8)
[31341.515455] cx25840 0-0044: Preferred audio mode: mono/language A
[31341.523377] cx25840 0-0044: IR Receiver:
[31341.523383] cx25840 0-0044: Enabled: no
[31341.523387] cx25840 0-0044: Demodulation from a carrier: disabled
[31341.523391] cx25840 0-0044: FIFO: disabled
[31341.523395] cx25840 0-0044: Pulse timers' start/stop trigger: disabled
[31341.523399] cx25840 0-0044: FIFO data on pulse timer overflow: overflow marker
[31341.523404] cx25840 0-0044: FIFO interrupt watermark: half full or greater
[31341.523407] cx25840 0-0044: Loopback mode: normal receive
[31341.523422] cx25840 0-0044: Max measurable pulse width: 318144512 us, 318144512000 ns
[31341.523427] cx25840 0-0044: Low pass filter: disabled
[31341.523431] cx25840 0-0044: Pulse width timer timed-out: no
[31341.523435] cx25840 0-0044: Pulse width timer time-out intr: enabled
[31341.523440] cx25840 0-0044: FIFO overrun: no
[31341.523443] cx25840 0-0044: FIFO overrun interrupt: enabled
[31341.523447] cx25840 0-0044: Busy: no
[31341.523451] cx25840 0-0044: FIFO service requested: no
[31341.523454] cx25840 0-0044: FIFO service request interrupt: enabled
[31341.523457] cx25840 0-0044: IR Transmitter:
[31341.523460] cx25840 0-0044: Enabled: no
[31341.523464] cx25840 0-0044: Modulation onto a carrier: disabled
[31341.523467] cx25840 0-0044: FIFO: disabled
[31341.523471] cx25840 0-0044: FIFO interrupt watermark: half full or less
[31341.523475] cx25840 0-0044: Carrier polarity: space:noburst mark:burst
[31341.523483] cx25840 0-0044: Max pulse width: 318144512 us, 318144512000 ns
[31341.523487] cx25840 0-0044: Busy: no
[31341.523490] cx25840 0-0044: FIFO service requested: yes
[31341.523493] cx25840 0-0044: FIFO service request interrupt: enabled
[31341.523509] cx25840 0-0044: Brightness: 128
[31341.523515] cx25840 0-0044: Contrast: 68
[31341.523522] cx25840 0-0044: Saturation: 64
[31341.523528] cx25840 0-0044: Hue: 0
[31341.523534] cx25840 0-0044: Volume: 62225
[31341.523541] cx25840 0-0044: Mute: false
[31341.523547] cx25840 0-0044: Balance: 0
[31341.523553] cx25840 0-0044: Bass: 0
[31341.523559] cx25840 0-0044: Treble: 0
[31341.523565] pvrusb2: cx2341x config:
[31341.523576] pvrusb2: Stream: MPEG-2 Program Stream
[31341.523582] pvrusb2: VBI Format: No VBI
[31341.523590] pvrusb2: Video: 720x480, 30 fps
[31341.523605] pvrusb2: Video: MPEG-2, 4x3, Variable Bitrate, 6000000, Peak 8000000
[31341.523610] pvrusb2: Video: GOP Size 12, 2 B-Frames, GOP Closure
[31341.523627] pvrusb2: Audio: 48 kHz, MPEG-1/2 Layer II, 224 kbps, Stereo, No Emphasis, No CRC
[31341.523636] pvrusb2: Spatial Filter: Manual, Luma 1D Horizontal, Chroma 1D Horizontal, 0
[31341.523641] pvrusb2: Temporal Filter: Manual, 8
[31341.523649] pvrusb2: Median Filter: Off, Luma [0, 255], Chroma [0, 255]
[31341.523663] pvrusb2_a driver: <ok> <init> <connected> <mode=analog>
[31341.523669] pvrusb2_a pipeline: <idle> <configok>
[31341.523681] pvrusb2_a worker: <decode:quiescent> <encode:virgin> <encode:waitok> <usb:stop> <pathway:ok>
[31341.523687] pvrusb2_a state: ready
[31341.523703] pvrusb2_a Hardware supported inputs: television, dtv, composite, s-video; allowed inputs: television, composite, s-video
[31341.523715] pvrusb2_a Bytes streamed=0 URBs: queued=0 idle=0 ready=0 processed=0 failed=0
[31341.523721] pvrusb2_a ir scheme: id=2 Zilog
[31341.523736] pvrusb2_a Associated v4l2-subdev drivers and I2C clients:
[31341.523739] pvrusb2_a cx25840: cx25840 @ 44
[31341.523743] pvrusb2_a tuner: tuner @ 42
[31341.523747] pvrusb2: ================== END STATUS CARD #0 ==================
The second line of the output reveals the cause of the problem.
For some strange reason, the default format for the cx25840 was changed from PAL-BDGHI to NTSC-M. (probably some default setting change between kernel 3.4 and 3.6/3.7)
This results in the behaviour described above. You still get a decent image but no sound.
To change the format back to PAL I added the following line to my script:
I hope some people use the to resolve the same problem... quicker... ;-)
Using the same script for many years I was surprised to notice that all the new recordings did not contain any sound.
For a moment I thought the device was broken but after re-trying with openSuSE 12.2 I concluded that it had to be a software error or setting.
Using the command
sudo v4l2-ctl -d /dev/video1 --log-statusI tried to do some debugging.
This is the output for openSuSE 12.2 where everything works as expected:
[ 1438.650407] pvrusb2: ================= START STATUS CARD #0 =================
[ 1438.652688] cx25840 9-0044: Video signal: present
[ 1438.652693] cx25840 9-0044: Detected format: PAL-BDGHI
[ 1438.652697] cx25840 9-0044: Specified standard: automatic detection
[ 1438.652701] cx25840 9-0044: Specified video input: Composite 7
[ 1438.652704] cx25840 9-0044: Specified audioclock freq: 48000 Hz
[ 1438.658092] cx25840 9-0044: Detected audio mode: mono
[ 1438.658101] cx25840 9-0044: Detected audio standard: A2-BG
[ 1438.658108] cx25840 9-0044: Audio microcontroller: running
[ 1438.658115] cx25840 9-0044: Configured audio standard: automatic detection
[ 1438.658122] cx25840 9-0044: Configured audio system: automatic standard and mode detection
[ 1438.658132] cx25840 9-0044: Specified audio input: Tuner (In8)
[ 1438.658140] cx25840 9-0044: Preferred audio mode: stereo
[ 1438.658149] cx25840 9-0044: Selected 65 MHz format: system DK
[ 1438.658155] cx25840 9-0044: Selected 45 MHz format: chroma
[ 1438.667850] cx25840 9-0044: IR Receiver:
[ 1438.667869] cx25840 9-0044: Enabled: no
[ 1438.667881] cx25840 9-0044: Demodulation from a carrier: disabled
[ 1438.667894] cx25840 9-0044: FIFO: disabled
[ 1438.667905] cx25840 9-0044: Pulse timers' start/stop trigger: disabled
[ 1438.667917] cx25840 9-0044: FIFO data on pulse timer overflow: overflow marker
[ 1438.667929] cx25840 9-0044: FIFO interrupt watermark: half full or greater
[ 1438.667941] cx25840 9-0044: Loopback mode: normal receive
[ 1438.667958] cx25840 9-0044: Max measurable pulse width: 318144512 us, 318144512000 ns
[ 1438.667974] cx25840 9-0044: Low pass filter: disabled
[ 1438.667990] cx25840 9-0044: Pulse width timer timed-out: no
[ 1438.668026] cx25840 9-0044: Pulse width timer time-out intr: enabled
[ 1438.668038] cx25840 9-0044: FIFO overrun: no
[ 1438.668048] cx25840 9-0044: FIFO overrun interrupt: enabled
[ 1438.668064] cx25840 9-0044: Busy: no
[ 1438.668076] cx25840 9-0044: FIFO service requested: no
[ 1438.668089] cx25840 9-0044: FIFO service request interrupt: enabled
[ 1438.668101] cx25840 9-0044: IR Transmitter:
[ 1438.668111] cx25840 9-0044: Enabled: no
[ 1438.668123] cx25840 9-0044: Modulation onto a carrier: disabled
[ 1438.668135] cx25840 9-0044: FIFO: disabled
[ 1438.668147] cx25840 9-0044: FIFO interrupt watermark: half full or less
[ 1438.668159] cx25840 9-0044: Carrier polarity: space:noburst mark:burst
[ 1438.668174] cx25840 9-0044: Max pulse width: 318144512 us, 318144512000 ns
[ 1438.668187] cx25840 9-0044: Busy: no
[ 1438.668200] cx25840 9-0044: FIFO service requested: yes
[ 1438.668211] cx25840 9-0044: FIFO service request interrupt: enabled
[ 1438.668227] cx25840 9-0044: Brightness: 128
[ 1438.668244] cx25840 9-0044: Contrast: 68
[ 1438.668262] cx25840 9-0044: Saturation: 64
[ 1438.668279] cx25840 9-0044: Hue: 0
[ 1438.668296] cx25840 9-0044: Volume: 62225
[ 1438.668313] cx25840 9-0044: Mute: false
[ 1438.668331] cx25840 9-0044: Balance: 0
[ 1438.668350] cx25840 9-0044: Bass: 0
[ 1438.668365] cx25840 9-0044: Treble: 0
[ 1438.668384] pvrusb2: cx2341x config:
[ 1438.668396] pvrusb2: Stream: MPEG-2 Program Stream
[ 1438.668412] pvrusb2: VBI Format: No VBI
[ 1438.668424] pvrusb2: Video: 720x480, 30 fps
[ 1438.668438] pvrusb2: Video: MPEG-2, 4x3, Variable Bitrate, 6000000, Peak 8000000
[ 1438.668460] pvrusb2: Video: GOP Size 12, 2 B-Frames, GOP Closure
[ 1438.668475] pvrusb2: Audio: 48 kHz, MPEG-1/2 Layer II, 224 kbps, Stereo, No Emphasis, No CRC
[ 1438.668494] pvrusb2: Spatial Filter: Manual, Luma 1D Horizontal, Chroma 1D Horizontal, 0
[ 1438.668509] pvrusb2: Temporal Filter: Manual, 8
[ 1438.668522] pvrusb2: Median Filter: Off, Luma [0, 255], Chroma [0, 255]
[ 1438.668541] pvrusb2_a driver: <ok> <init> <connected> <mode=analog>
[ 1438.668555] pvrusb2_a pipeline: <idle> <configok>
[ 1438.668567] pvrusb2_a worker: <decode:quiescent> <encode:virgin> <encode:waitok> <usb:stop> <pathway:ok>
[ 1438.668581] pvrusb2_a state: ready
[ 1438.668597] pvrusb2_a Hardware supported inputs: television, dtv, composite, s-video; allowed inputs: television, composite, s-video
[ 1438.668622] pvrusb2_a Bytes streamed=0 URBs: queued=0 idle=0 ready=0 processed=0 failed=0
[ 1438.668636] pvrusb2_a ir scheme: id=2 Zilog
[ 1438.668650] pvrusb2_a Associated v4l2-subdev drivers and I2C clients:
[ 1438.668664] pvrusb2_a cx25840: cx25840 @ 44
[ 1438.668676] pvrusb2_a tuner: tuner @ 42
[ 1438.668686] pvrusb2: ================== END STATUS CARD #0 ==================
And this is the output for openSuSE 12.3... let's look for the difference!
[31341.497675] pvrusb2: ================= START STATUS CARD #0 =================
[31341.500021] cx25840 0-0044: Video signal: not present
[31341.500041] cx25840 0-0044: Detected format: NTSC-M
[31341.500053] cx25840 0-0044: Specified standard: automatic detection
[31341.500065] cx25840 0-0044: Specified video input: Composite 7
[31341.500077] cx25840 0-0044: Specified audioclock freq: 48000 Hz
[31341.515412] cx25840 0-0044: Detected audio mode: forced mode
[31341.515423] cx25840 0-0044: Detected audio standard: forced audio standard
[31341.515429] cx25840 0-0044: Audio microcontroller: detecting
[31341.515436] cx25840 0-0044: Configured audio standard: undefined
[31341.515443] cx25840 0-0044: Configured audio mode: MONO1 (LANGUAGE A/Mono L+R channel for BTSC, EIAJ, A2)
[31341.515449] cx25840 0-0044: Specified audio input: Tuner (In8)
[31341.515455] cx25840 0-0044: Preferred audio mode: mono/language A
[31341.523377] cx25840 0-0044: IR Receiver:
[31341.523383] cx25840 0-0044: Enabled: no
[31341.523387] cx25840 0-0044: Demodulation from a carrier: disabled
[31341.523391] cx25840 0-0044: FIFO: disabled
[31341.523395] cx25840 0-0044: Pulse timers' start/stop trigger: disabled
[31341.523399] cx25840 0-0044: FIFO data on pulse timer overflow: overflow marker
[31341.523404] cx25840 0-0044: FIFO interrupt watermark: half full or greater
[31341.523407] cx25840 0-0044: Loopback mode: normal receive
[31341.523422] cx25840 0-0044: Max measurable pulse width: 318144512 us, 318144512000 ns
[31341.523427] cx25840 0-0044: Low pass filter: disabled
[31341.523431] cx25840 0-0044: Pulse width timer timed-out: no
[31341.523435] cx25840 0-0044: Pulse width timer time-out intr: enabled
[31341.523440] cx25840 0-0044: FIFO overrun: no
[31341.523443] cx25840 0-0044: FIFO overrun interrupt: enabled
[31341.523447] cx25840 0-0044: Busy: no
[31341.523451] cx25840 0-0044: FIFO service requested: no
[31341.523454] cx25840 0-0044: FIFO service request interrupt: enabled
[31341.523457] cx25840 0-0044: IR Transmitter:
[31341.523460] cx25840 0-0044: Enabled: no
[31341.523464] cx25840 0-0044: Modulation onto a carrier: disabled
[31341.523467] cx25840 0-0044: FIFO: disabled
[31341.523471] cx25840 0-0044: FIFO interrupt watermark: half full or less
[31341.523475] cx25840 0-0044: Carrier polarity: space:noburst mark:burst
[31341.523483] cx25840 0-0044: Max pulse width: 318144512 us, 318144512000 ns
[31341.523487] cx25840 0-0044: Busy: no
[31341.523490] cx25840 0-0044: FIFO service requested: yes
[31341.523493] cx25840 0-0044: FIFO service request interrupt: enabled
[31341.523509] cx25840 0-0044: Brightness: 128
[31341.523515] cx25840 0-0044: Contrast: 68
[31341.523522] cx25840 0-0044: Saturation: 64
[31341.523528] cx25840 0-0044: Hue: 0
[31341.523534] cx25840 0-0044: Volume: 62225
[31341.523541] cx25840 0-0044: Mute: false
[31341.523547] cx25840 0-0044: Balance: 0
[31341.523553] cx25840 0-0044: Bass: 0
[31341.523559] cx25840 0-0044: Treble: 0
[31341.523565] pvrusb2: cx2341x config:
[31341.523576] pvrusb2: Stream: MPEG-2 Program Stream
[31341.523582] pvrusb2: VBI Format: No VBI
[31341.523590] pvrusb2: Video: 720x480, 30 fps
[31341.523605] pvrusb2: Video: MPEG-2, 4x3, Variable Bitrate, 6000000, Peak 8000000
[31341.523610] pvrusb2: Video: GOP Size 12, 2 B-Frames, GOP Closure
[31341.523627] pvrusb2: Audio: 48 kHz, MPEG-1/2 Layer II, 224 kbps, Stereo, No Emphasis, No CRC
[31341.523636] pvrusb2: Spatial Filter: Manual, Luma 1D Horizontal, Chroma 1D Horizontal, 0
[31341.523641] pvrusb2: Temporal Filter: Manual, 8
[31341.523649] pvrusb2: Median Filter: Off, Luma [0, 255], Chroma [0, 255]
[31341.523663] pvrusb2_a driver: <ok> <init> <connected> <mode=analog>
[31341.523669] pvrusb2_a pipeline: <idle> <configok>
[31341.523681] pvrusb2_a worker: <decode:quiescent> <encode:virgin> <encode:waitok> <usb:stop> <pathway:ok>
[31341.523687] pvrusb2_a state: ready
[31341.523703] pvrusb2_a Hardware supported inputs: television, dtv, composite, s-video; allowed inputs: television, composite, s-video
[31341.523715] pvrusb2_a Bytes streamed=0 URBs: queued=0 idle=0 ready=0 processed=0 failed=0
[31341.523721] pvrusb2_a ir scheme: id=2 Zilog
[31341.523736] pvrusb2_a Associated v4l2-subdev drivers and I2C clients:
[31341.523739] pvrusb2_a cx25840: cx25840 @ 44
[31341.523743] pvrusb2_a tuner: tuner @ 42
[31341.523747] pvrusb2: ================== END STATUS CARD #0 ==================
The second line of the output reveals the cause of the problem.
For some strange reason, the default format for the cx25840 was changed from PAL-BDGHI to NTSC-M. (probably some default setting change between kernel 3.4 and 3.6/3.7)
This results in the behaviour described above. You still get a decent image but no sound.
To change the format back to PAL I added the following line to my script:
v4l2-ctl -s pal-BSeems like an unimportant post but when looking for the problem I found a lot of people with a similar problem but no fix.
I hope some people use the to resolve the same problem... quicker... ;-)
Tuesday, March 19, 2013
Installing openSuSE 12.3 on an external USB HDD
Another openSuSE version... another post about installing it...
That means that the disk was detected while booting from DVD as the SECOND DISK.
You will have to change the proposed Grub configuration (by clicking on the BOOTING link)
First, make sure you select GRUB and not GRUB2 (doesn't play nice with this setup)
This time I used the 32-bit DVD version but the procedure should also work for the live CD's.
Make sure you uncheck the "use automatic configuration" box when prompted.
I created a root partition on the external disk and formatted it with EXT4.
As you can see on the screenshot it is called /dev/sdb1
As you can see on the screenshot it is called /dev/sdb1
That means that the disk was detected while booting from DVD as the SECOND DISK.
However, when you boot from USB, the BIOS will report the USB disk as the FIRST disk.
This is important for the Grub configuration.
You will have to change the proposed Grub configuration (by clicking on the BOOTING link)
First, make sure you select GRUB and not GRUB2 (doesn't play nice with this setup)
Then make sure you only tick the box: "Boot from root partition"
And finally open the advanced section and move the USB disk to the top so that the disk order represents the BIOS order when booting from USB.
All this should result in the following summary:
Now resume your installation as you would normally do and enjoy this new release...
Sunday, January 27, 2013
Recover from GRUB blinking cursor
I tried to upgrade to the latest standard kernel but something went wrong and after the power cycle the system would not boot any longer.
Even the grub menu did not display. (Graphical nor text)
I just had a black display with the word GRUB and a blinking cursor.
This is how I managed to repair the system.
Even the grub menu did not display. (Graphical nor text)
I just had a black display with the word GRUB and a blinking cursor.
This is how I managed to repair the system.
- Boot from the DVD and choose the "Rescue system" option
- Login as root (empty password)
- mount /dev/sda2 /mnt (mount your root partition)
- mount /dev/sda1 /mnt/boot (mount the boot partition, if separate)
- mount --bind /dev /mnt/dev (hardware mounting)
- chroot /mnt (chroot into the system)
- mount /proc
- mount /sys
- Run YaST - System - Boot Loader
- Replace "Grub2" with "grub" and default options.
- Reboot...
This fixed it for me...
I did try to repair grub2 first but never got past the black screen...
Subscribe to:
Posts (Atom)